2014-09-23-Tue
Overriding |
class AA { void callMe() { System.out.println("class AA's callMe"); } } class BB extends AA { void callMe() { System.out.println("class BB's callMe"); } } class CC extends BB { void callMe() { System.out.println("class CC's callMe"); } } class Overriding { public static void main(String[] args) { AA ref = new AA(); ref.callMe(); ref=new BB(); // AA타입 ref에 BB객체 선언 ~ 다형성 원리로 가능 ref.callMe(); ref = new CC(); ref.callMe(); } } |
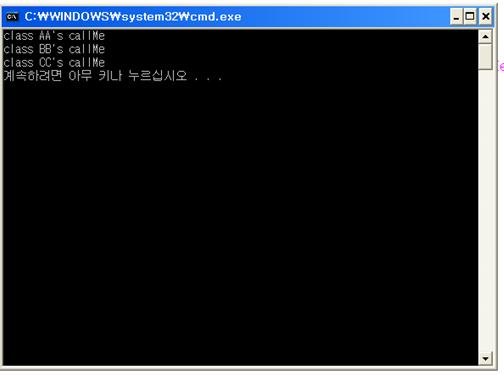 |
- Overriding(재정의)
- Polymophism(다형성)
* 깨알 톡 *
무진장 피곤 졸림.........그분이 오시려나보다.......
오늘 네트워크 복습은 무싄일이 있더라도 해야한당
고시프 예습도 해야하ㅡㄴㄴ데
개 피곤해~~~~~~~~~~~
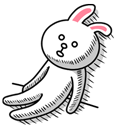
동적 바인딩
:: callMe()가 여러개 있는데 연결되는것은 동적으로 이루어진다.
컴파일러 상에서는 알 수 없지만 실행시 결정
정적 바인딩
:: 대부분의 코드는 거진 정적 바인딩 ~ 컴파일 시 결정
|
AA ref = new AA(); ref.callMe(); //ref=new BB(); // AA타입 ref에 BB객체 선언 ~ 다형성 원리로 가능 ref.callMe(); ref = new CC(); ref.callMe(); |
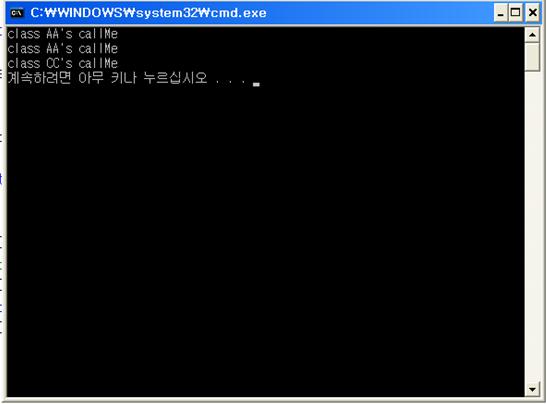 |
|
class AA { int x =1; void callMe() { System.out.println("class AA's callMe"); } } class BB extends AA { int x=2; void callMe() { System.out.println("class BB's callMe"); } } class CC extends BB { int x=3; void callMe() { System.out.println("class CC's callMe"); } } class Overriding { public static void main(String[] args) { AA ref = new AA(); ref.callMe(); System.out.format("x is %d\n",ref.x); ref=new BB(); ref.callMe(); System.out.format("x is %d\n",ref.x); ref = new CC(); ref.callMe(); System.out.format("x is %d\n",ref.x); } } |
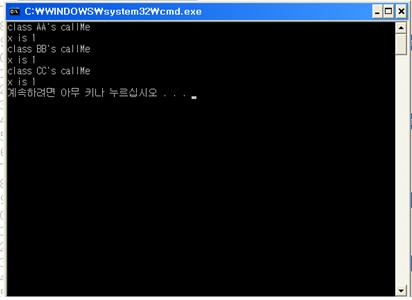 |
타입이 맞는 변수형만 사용해야한다.
|
class AA { int x =1; void callMe() { System.out.println("class AA's callMe"); } } class BB extends AA { int x=2; void callMe() { System.out.println("class BB's callMe"); } } class CC extends BB { int x=3; void callMe() { System.out.println("class CC's callMe"); } } class Overriding { public static void main(String[] args) { AA ref = new AA(); ref.callMe(); System.out.format("x is %d\n",ref.x); ref=new BB(); ref.callMe(); System.out.format("x is %d\n",ref.x); ref = new CC(); ref.callMe(); System.out.format("x is %d\n",ref.x); BB refB = new CC(); ref.callMe(); System.out.format("x is %d\n",refB.x); } } |
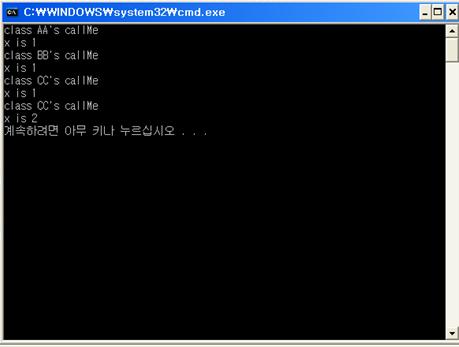 |
더워~~~~~~!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!하앍 하앍ㅠㅠㅠㅠㅠㅠㅠㅠㅠ
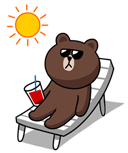
|
class Product { int price; static int totalSale=0; Product(int pr){price=pr;} } class Tv extends Product { Tv(){super(100);} public String toString(){return "Tv Purchased:";} } class Computer extends Product { Computer(){super(200);} public String toString(){return "Computer Purchased";} } class Audio extends Product { Audio(){super(150);} public String toString(){return "Audio Purchased";} } class Buyer { String name; int money; Product [] cart =new Product[10]; int count=0; Buyer(String name, int money) { this.name=name; this.money=money; } //메서드 오버로딩 //-> Tv대신 product 따로 설정 void buy(Product p) { money=money-p.price; cart[count++]=p; Product.totalSale+=p.price;} //void buy(Tv t){money=money-t.price;} //void buy(Computer c){money-=c.price;} //void buy(Audio a){money-=a.price;} void printProduct(){ int sum=0; for(int i=0;i<count;i++) { System.out.println(cart[i]); sum+=cart[i].price; } System.out.println("Sum : "+ sum); } } class Pr07Ploy { public static void main(String[] args) { Tv tv1=new Tv(); Computer comp1=new Computer(); Audio audio1=new Audio(); Buyer b1=new Buyer("Chul-soo",1000); b1.buy(tv1); b1.buy(comp1); //System.out.println(tv1); System.out.println(b1.name+":Money left="+b1.money ); /* Product p1 = new Tv(); Product p2 = new Computer(); b1.buy(p1); b1.buy(p2); */ Product[] p = new Product[3]; p[0]=new Tv(); p[1]=new Computer(); for( int i=0;i<2;i++){ b1.buy(p[i]); } System.out.println(b1.name+":Money left="+b1.money ); b1.printProduct(); Buyer b2 = new Buyer("YoungHee",200); b2.buy(tv1);b2.buy(audio1); b2.printProduct(); System.out.println("Total sale :: "+Product.totalSale ); } } |
|
댓글